Example: Circuit SystemY#
from opendssdirect import dss
# If you downloaded just the notebook, remember to update the path to the DSS script below
dss('Redirect "../../tests/data/13Bus/IEEE13Nodeckt.dss"')
The function dss.YMatrix.getYsparse()
returns the vectors that form the compressed sparse representation of the matrix. This is the preferred usage for large system.
We recommend using scipy.sparse
for this usage:
from scipy.sparse import csc_matrix
systemY = csc_matrix(dss.YMatrix.getYsparse())
systemY
<41x41 sparse matrix of type '<class 'numpy.complex128'>'
with 267 stored elements in Compressed Sparse Column format>
print(systemY)
(0, 0) (0.8178822742754986-4.636999108384914j)
(1, 0) (-0.13055025449836521+1.4833269062112926j)
(2, 0) (-0.13055025449836524+1.4833269062112926j)
(3, 0) (-9.283352668986776+74.26682135189421j)
(4, 0) (9.283352668986776-74.26682135189421j)
(0, 1) (-0.13055025449836521+1.4833269062112926j)
(1, 1) (0.8178822742754985-4.636999108384914j)
(2, 1) (-0.13055025449836524+1.4833269062112926j)
(4, 1) (-9.283352668986776+74.26682135189421j)
(5, 1) (9.283352668986776-74.26682135189421j)
(0, 2) (-0.13055025449836524+1.4833269062112926j)
(1, 2) (-0.13055025449836524+1.4833269062112926j)
(2, 2) (0.8178822742754985-4.636999108384913j)
(3, 2) (9.283352668986776-74.26682135189421j)
(5, 2) (-9.283352668986776+74.26682135189421j)
(0, 3) (-9.283352668986776+74.26682135189421j)
(2, 3) (9.283352668986776-74.26682135189421j)
(3, 3) (1890.6787348910132-5002.165990528294j)
(6, 3) (-1369.165023011177+1369.165023011177j)
(0, 4) (9.283352668986776-74.26682135189421j)
(1, 4) (-9.283352668986776+74.26682135189421j)
(4, 4) (1890.6787348910132-5002.165990528294j)
(7, 4) (-1393.9089692101743+1393.9089692101743j)
(1, 5) (9.283352668986776-74.26682135189421j)
(2, 5) (-9.283352668986776+74.26682135189421j)
: :
(35, 36) (-0.2526096623056667+1.3934005374671847j)
(36, 36) (1.7734368716962685-5.901187416512638j)
(18, 37) (-3.8523736320183444+1.598948297622844j)
(19, 37) (2.407733520011465-0.9993426967242526j)
(20, 37) (-7.175068362002635+6.675422271119008j)
(32, 37) (1.4446401120068793-0.5996056180345513j)
(33, 37) (-4.3050410172015825+4.0052533626714055j)
(37, 37) (11.480109379204219-10.680675553822601j)
(16, 38) (-10000000+0j)
(21, 38) (-2.4286016759142925+3.079395289523383j)
(22, 38) (-2.428601675914292+3.079395289523384j)
(23, 38) (2.428601675914292-3.079395289523384j)
(24, 38) (-11.546745157318384+8.197439395398543j)
(25, 38) (2.4286016759142925-3.079395289523383j)
(38, 38) (10000011.546745157-8.197432541942769j)
(15, 39) (-7.175068362002635+6.675422271119008j)
(17, 39) (2.407733520011465-0.9993426967242526j)
(27, 39) (-4.291089932048226+1.6378059450141607j)
(39, 39) (11.46615829405086-8.313221446001002j)
(40, 39) (-2.407733520011465+0.9993426902982676j)
(15, 40) (2.407733520011465-0.9993426967242526j)
(17, 40) (-7.224938066978001+6.644943442253672j)
(26, 40) (-6.5300017720164165+6.619904745555312j)
(39, 40) (-2.407733520011465+0.9993426902982676j)
(40, 40) (13.754939838994417-13.264848127833126j)
If you have matplotlib
installed, you can plot it with spy
:
from matplotlib import pyplot as plt
plt.spy(systemY)
<matplotlib.lines.Line2D at 0x7ff3bb91c080>
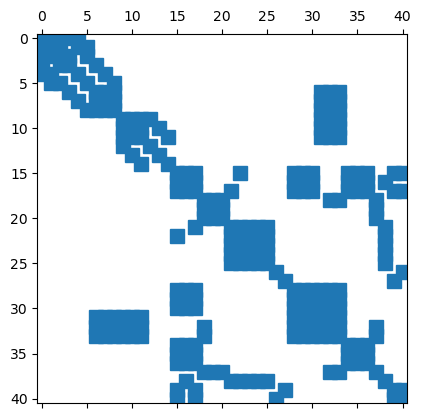
Although not recommended, you can also get the whole dense system Y matrix as below, just like in the official OpenDSS COM implementation. This is kept for compatibility with the official implementation. It is useful to quickly analyze very small circuits without installing extra Python packages.
dss.Circuit.SystemY() # avoid, this is a dense matrix (memory intensive)
[0.8178822742754986,
-4.636999108384914,
-0.13055025449836521,
1.4833269062112926,
-0.13055025449836524,
1.4833269062112926,
-9.283352668986776,
74.26682135189421,
9.283352668986776,
-74.26682135189421,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
-0.13055025449836521,
1.4833269062112926,
0.8178822742754985,
-4.636999108384914,
-0.13055025449836524,
1.4833269062112926,
0.0,
0.0,
-9.283352668986776,
74.26682135189421,
9.283352668986776,
-74.26682135189421,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
-0.13055025449836524,
1.4833269062112926,
-0.13055025449836524,
1.4833269062112926,
0.8178822742754985,
-4.636999108384913,
9.283352668986776,
-74.26682135189421,
0.0,
0.0,
-9.283352668986776,
74.26682135189421,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
-9.283352668986776,
74.26682135189421,
0.0,
0.0,
9.283352668986776,
-74.26682135189421,
1890.6787348910132,
-5002.165990528294,
0.0,
0.0,
0.0,
0.0,
-1369.165023011177,
1369.165023011177,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
9.283352668986776,
-74.26682135189421,
-9.283352668986776,
74.26682135189421,
0.0,
0.0,
0.0,
0.0,
1890.6787348910132,
-5002.165990528294,
0.0,
0.0,
0.0,
0.0,
-1393.9089692101743,
1393.9089692101743,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
9.283352668986776,
-74.26682135189421,
-9.283352668986776,
74.26682135189421,
0.0,
0.0,
0.0,
0.0,
1890.6787348910132,
-5002.165990528294,
0.0,
0.0,
0.0,
0.0,
-1369.165023011177,
1369.165023011177,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
-1369.165023011177,
1369.165023011177,
0.0,
0.0,
0.0,
0.0,
1297.3960314120725,
-1299.5515100351274,
-0.485857546024989,
1.22029123063551,
-0.26605890984743125,
0.9122168082594669,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
-1.1451220523783028,
3.3006007307348617,
0.485857546024989,
-1.2202912734754099,
0.26605890984743125,
-0.9122168510993668,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
-1393.9089692101743,
1393.9089692101743,
0.0,
0.0,
-0.485857546024989,
1.22029123063551,
1344.5294284920049,
-1346.6543624309695,
-0.12630483115283336,
0.6967002366036674,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.485857546024989,
-1.2202912734754099,
-1.0027111809933547,
3.1276451752596324,
0.12630483115283336,
-0.6967002794435673,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
-1369.165023011177,
1369.165023011177,
-0.26605890984743125,
0.9122168082594669,
-0.12630483115283336,
0.6967002366036674,
1297.1376277955421,
-1299.201503062629,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.26605890984743125,
-0.9122168510993668,
0.12630483115283336,
-0.6967002794435673,
-0.8867184358481343,
2.9505937582362023,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
6.174634422355675,
-8.24293119603154,
-1.495155595100418,
1.5059091102776052,
-2.1394799752143716,
1.6901170943579362,
-5.286769772134453,
9.612308676608098,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
-5.564622525570931,
7.133818691956606,
1.495155595100418,
-1.5059091209875801,
2.1394799752143716,
-1.6901171050679111,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
-1.495155595100418,
1.5059091102776052,
5.5185532759822955,
-8.172535006552001,
-1.0880240059003325,
1.3823966977507467,
0.0,
0.0,
-5.286769772134453,
9.612308676608098,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
1.495155595100418,
-1.5059091209875801,
-4.908541379197551,
7.063422502477067,
1.0880240059003325,
-1.3823967084607216,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
-2.1394799752143716,
1.6901170943579362,
-1.0880240059003325,
1.3823966977507467,
5.799215580883693,
-8.194725900906395,
0.0,
0.0,
0.0,
0.0,
-5.286769772134453,
9.612308676608098,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
2.1394799752143716,
-1.6901171050679111,
1.0880240059003325,
-1.3823967084607216,
-5.189203684098949,
7.085613396831461,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
...]